Saturday 21 April 2012
Extention Methods In .Net
You want to create Method inside any of the system class or your custom class without creating a new derived type, recompiling, or otherwise modifying the original type/class.
Here is solution ...
Create extention method.
Extension methods enable you to "add" methods to existing types without creating a new derived type, recompiling, or otherwise modifying the original type. Extension methods are a special kind of static method, but they are called as if they were instance methods on the extended type. For client code written in C# and Visual Basic, there is no apparent difference between calling an extension method and the methods that are actually defined in a type.
See below screen shot :
you can try it with string.
As its not possible to override string class and its methods, the reasonbeing that String is defined to be a Sealed class. You can extend it using extention method.
Refer this MSDN artical for more details: http://msdn.microsoft.com/en-us/library/bb383977.aspx.
In this article we will be seeing how to change
the Advanced Settings for Document library in SharePoint 2010 using PowerShell
and c#.
Go to Document Library => Library Settings => General Settings =>Advanced Settings.
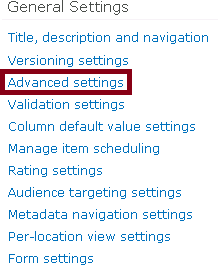
Using C#:using (SPSite site = new SPSite("http://serverName:1111/"))
{
using (SPWeb web = site.RootWeb)
{
SPList docLibrary=web.Lists["Doc Library"];
Go to Document Library => Library Settings => General Settings =>Advanced Settings.
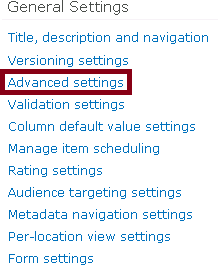
Using C#:using (SPSite site = new SPSite("http://serverName:1111/"))
{
using (SPWeb web = site.RootWeb)
{
SPList docLibrary=web.Lists["Doc Library"];
// Change the advanced
settings
// Update the changes
docLibrary.Update();
}
}
Using PowerShell
$site=Get-SPSite "http://serverName:1111/"
$web=$site.RootWeb
$docLibrary =$web.Lists["Doc Library"]
# Change the advanced settings
$docLibrary.Update()
Content Types:
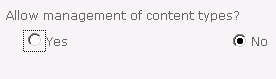
C#:docLibrary.ContentTypesEnabled = false;
PowerShell:
$docLibrary.ContentTypesEnabled = $false
Opening Documents in the Browser: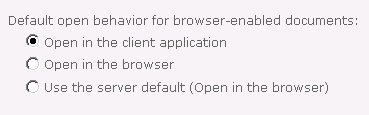
C#:// Open in the client applicationdocLibrary.DefaultItemOpen = DefaultItemOpen.PreferClient;
}
}
Using PowerShell
$site=Get-SPSite "http://serverName:1111/"
$web=$site.RootWeb
$docLibrary =$web.Lists["Doc Library"]
# Change the advanced settings
$docLibrary.Update()
Content Types:
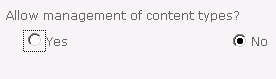
C#:docLibrary.ContentTypesEnabled = false;
PowerShell:
$docLibrary.ContentTypesEnabled = $false
Opening Documents in the Browser:
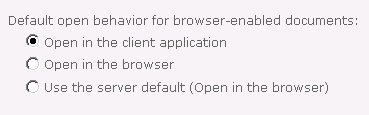
C#:// Open in the client applicationdocLibrary.DefaultItemOpen = DefaultItemOpen.PreferClient;
//
Open in the browserdocLibrary.DefaultItemOpen
= DefaultItemOpen.Browser;
//
Use the server defaultdocLibrary.DefaultItemOpenUseListSetting
= false;
PowerShell:
#Open in the client application
$docLibrary.DefaultItemOpen = "PreferClient"
#Open in the browser
$docLibrary.DefaultItemOpen = "Browser"
#Use the server default
$docLibrary.DefaultItemOpenUseListSetting = $false
Custom Send To Destination:
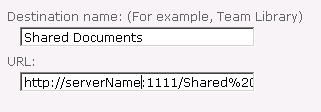
C#:docLibrary.SendToLocationName = "Shared Documents";
docLibrary.SendToLocationUrl = "http://serverName:1111/Shared%20Documents/";
PowerShell:
$docLibrary.SendToLocationName = "Shared Documents";
$docLibrary.SendToLocationUrl = "http://serverName:1111/Shared%20Documents/";
Folders:
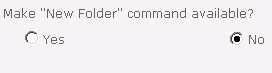
C#:docLibrary.EnableFolderCreation = false;
PowerShell:$docLibrary.EnableFolderCreation = $false
Search:

C#:docLibrary.NoCrawl = true;
PowerShell:$docLibrary.NoCrawl = $true
Offline Client Availability:
C#:docLibrary.ExcludeFromOfflineClient = true;
PowerShell:
$docLibrary.ExcludeFromOfflineClient = $true
Site Assets Library:

C#:
docLibrary.IsSiteAssetsLibrary = false;
PowerShell:
$docLibrary.IsSiteAssetsLibrary = $false
Datasheet:
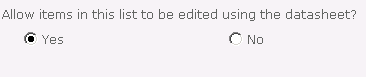
C#:
docLibrary.DisableGridEditing = true;
PowerShell:
$docLibrary.DisableGridEditing = $true
Dialogs:
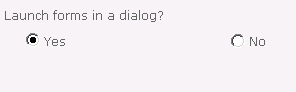
C#:
docLibrary.NavigateForFormsPages = true;
PowerShell:
$docLibrary.NavigateForFormsPages = $true
PowerShell:
#Open in the client application
$docLibrary.DefaultItemOpen = "PreferClient"
#Open in the browser
$docLibrary.DefaultItemOpen = "Browser"
#Use the server default
$docLibrary.DefaultItemOpenUseListSetting = $false
Custom Send To Destination:
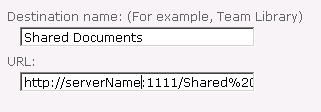
C#:docLibrary.SendToLocationName = "Shared Documents";
docLibrary.SendToLocationUrl = "http://serverName:1111/Shared%20Documents/";
PowerShell:
$docLibrary.SendToLocationName = "Shared Documents";
$docLibrary.SendToLocationUrl = "http://serverName:1111/Shared%20Documents/";
Folders:
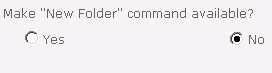
C#:docLibrary.EnableFolderCreation = false;
PowerShell:$docLibrary.EnableFolderCreation = $false
Search:

C#:docLibrary.NoCrawl = true;
PowerShell:$docLibrary.NoCrawl = $true
Offline Client Availability:

C#:docLibrary.ExcludeFromOfflineClient = true;
PowerShell:
$docLibrary.ExcludeFromOfflineClient = $true
Site Assets Library:

C#:
docLibrary.IsSiteAssetsLibrary = false;
PowerShell:
$docLibrary.IsSiteAssetsLibrary = $false
Datasheet:
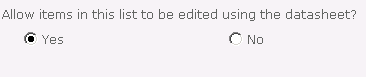
C#:
docLibrary.DisableGridEditing = true;
PowerShell:
$docLibrary.DisableGridEditing = $true
Dialogs:
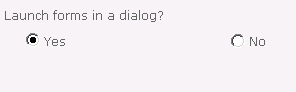
C#:
docLibrary.NavigateForFormsPages = true;
PowerShell:
$docLibrary.NavigateForFormsPages = $true
What is Content in SharePoint?
Content comprises of documents and list items. Content does not include list or library itself.List or library helps user to organize the content.
Content is categorized into : structured and unstructured content.
Structured content:
Content which separates storage of its content from its display. e.g. List Items can be sorted/filtered and viewed in List web part or data row in a SQL data table.
Unstructured Content:
Content which cannot be viewed separately the format in which they are stored. e.g. Word document cannot be viewed without the MS Office Word.
Subscribe to:
Posts (Atom)